PROJECT: FlashCard Pro
Overview
FlashCardPro is a program to manage flash cards each with three properties; front, back and evaluation.
The simplest form of a flash card is a FrontBackCard
where the front and back are texts and evaluation
is checking that the user’s input is the same as back.
The app also has a review mode where users can test themselves with the cards they have created.
Cards can also easily be shared via a json file per deck of cards.
This project is implemented from scratch while learning the ropes of design patterns and software architecture.
Code Contribution
Major Contribution
-
Came up with initial structure for the model of abstract flash cards #52
-
Came up with high level design for feedback loop of processing input and gui interaction #52 Responder Responses ResponseFunc State
-
Implemented
FileReadWrite
library used by the team to store and read files in a centralized location. #63 FileReadWrite -
Implemented JSON parsing algorithm from scratch that takes a string input and creates
JsonValue
objects that can be interpreted in java to reconstruct objects that it represents. #63 All JSON Parsing -
Implemented a cli that reads keystrokes and based on that action determines how to edit and present text for the user. It is to emulate a terminal window. It also has multiline editing capabilities (a mini text editor), all implemented using purely javafx
KeyEvent
and timer. #277 #279 #284 #336 #361 #372
Documentation Contribution
Miscellaneous Documentation
Wrote a tutorial for the team to understand Responses
at its most basic form
Storage component
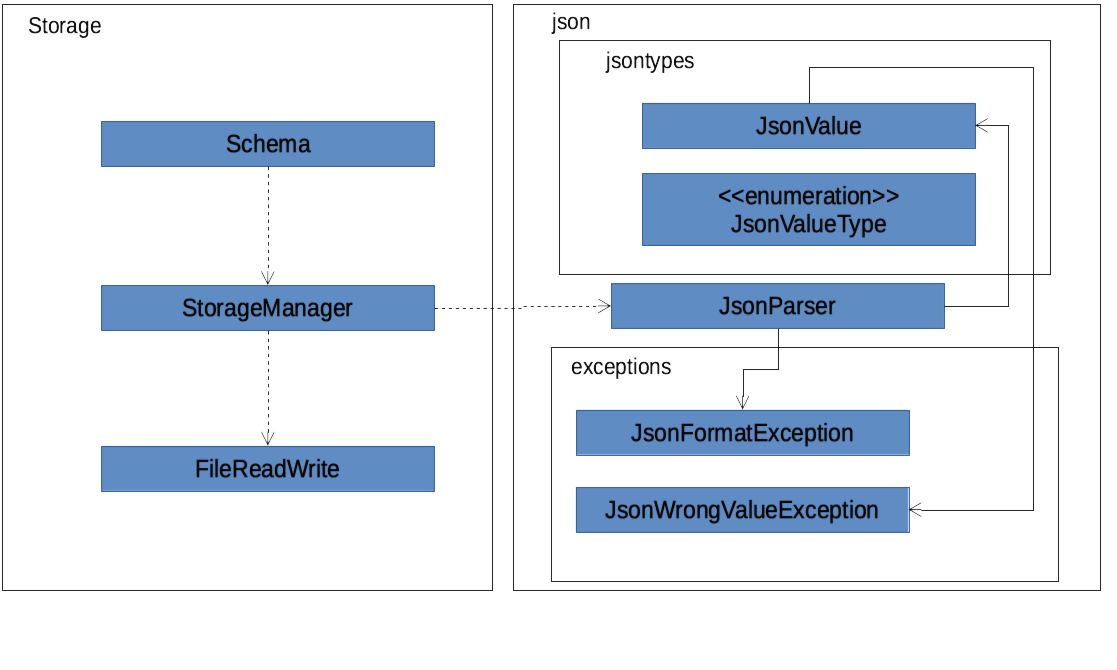
API : StorageManager.java
API : JsonParser.java
API : FileReadWrite.java
The Storage
component,
-
interface to save deck by calling
FileReadWrite
-
interface to load deck by calling
FileReadWrite
, send string toJsonParser
and creating deck objects fromJsonValue
The JsonParser
component,
-
takes any string of JSON format and returns a
JsonValue
The FileReadWrite
component,
-
resolves root directory for app save data
-
interface for user to provide their custom root directory
-
writes file and creates path directories if none
Implementation
The parsing is done by JsonParser
.
It takes a string and attempts to read it as one of a JsonValueTypes
and create its corresponding object wrapped in JsonValue
.
Since Objects and Arrays can recursively contain a json value, their contents are also read, created and wrapped.
-
JsonParser#parseJsonInput()
— constructs theJsonValue
object from a string input
Given below is the overview activity diagram of parsing a json string input.
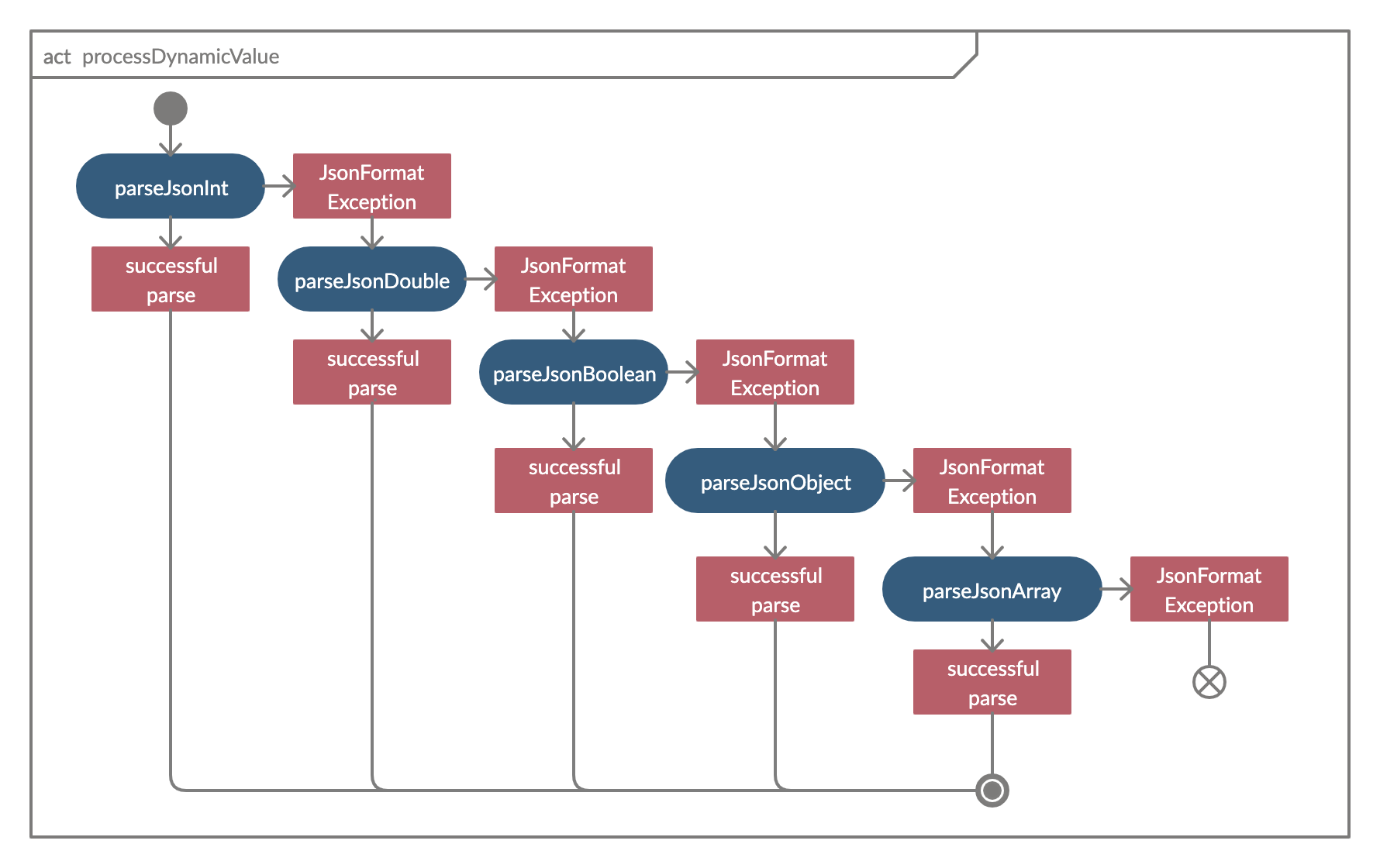
It will first be tested to see if its an integer and if it fails it will be tested for a double. Consequently, boolean, string, json object, json array.
Json object values are themselves json values thus the activity diagram is called recursively Likewise for json array values.
If all parsing types fail, there must be an error with the string input, thus a JsonFormatException is thrown. |
Design Considerations
The JsonParser is designed to be a utility class with a pure function without any mutation of state. Thus it should be self contained within a single function call.
Having that we are using the regex approach to parsing, some common parsing and regex creation methods are stored in the following class:
API : RegexUtil.java
RegexUtil#commandFormatRegex` creates a regex that starts with the command
argument and lookaheads
for the elements of args
. Thus a regex for the input create front/asd back/dsa
can be created with
commandFormatRegex("create", new String[]{"front/", "back/"});
.
RegexUtil#parseCommandFormat
is an algorithm that parses user input and returns the resulting values
from the input. Following the previous example, parsing the input will create an arraylist of two arraylists.
The first arraylist contains one element "asd"
and the second arraylist contains one element "dsa"
;
parallel to the arguments of the input.
User Guide
Command History
-
After keying in a command, press up and down arrow keys to navigate your command history.
-
If in multiline editing mode, press (Ctrl+d) to submit the multiline text
-
In single line mode use (Ctrl+c) to clear the line
-
Press (Ctrl+v) to paste clipboard content. If in multiline mode it will paste as per normal. In singleline mode each line will be evaluated.